参数回调 OnSetParametersCallbackHandle
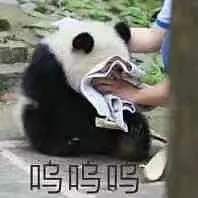
OnSetParametersCallbackHandle 在于foxy版本添加,dashing中还尚未支持。
在foxy版本中,也添加了返回此类型的回调函数,
1 | /// Add a callback for when parameters are being set. |
这是用于替代dashing版本中的set_on_parameters_set_callback
1 | /// Register a callback to be called anytime a parameter is about to be changed. |
Comments