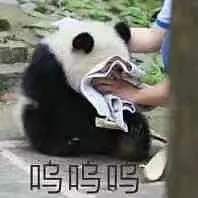
源https://navigation.ros.org/plugin_tutorials/docs/writing_new_nav2planner_plugin.html
创建一个新的Planner插件
该教程会指导我们如何制作一个走直线的planner
前置要求
- ROS2
- Nav2
- Gazebo
- turtlebot3
步骤一:创建一个新的Planner插件
我们会创建一个简单的走直线的Planner插件,代码在navigation_tutorials 仓库中,名字为nav2_straightline_planner。我们的插件继承于nav2_core::GlobalPlanner
. 基类提供了五个纯虚函数,这个插件会被Planner Server调用来计算轨迹
Virtual method | Method description | Requires override? |
---|---|---|
configure() | Method is called at when planner server enters on_configure state. Ideally this methods should perform declarations of ROS parameters and initialization of planner’s member variables. This method takes 4 input params: shared pointer to parent node, planner name, tf buffer pointer and shared pointer to costmap. | Yes |
activate() | Method is called when planner server enters on_activate state. Ideally this method should implement operations which are neccessary before planner goes to an active state. | Yes |
deactivate() | Method is called when planner server enters on_deactivate state. Ideally this method should implement operations which are neccessary before planner goes to an inactive state. | Yes |
cleanup() | Method is called when planner server goes to on_cleanup state. Ideally this method should clean up resoures which are created for the planner. | Yes |
createPlan() | Method is called when planner server demands a global plan for specified start and goal pose. This method returns nav_msgs::msg::Path carrying global plan. This method takes 2 input parmas: start pose and goal pose. | Yes |
在本教程中,我们会利用StraightLine::configure() 和 StraightLine::createPlan()来创建直线planner.
在Planner中,configure() 方法必须要设置好成员变量
1 | node_ = parent; |
name_ + “.interpolation_resolution” 的值可以从ROS 参数系统中获得,并把他赋值给我们定义的成员变量,interpolation_resolution。Nav2可以同时加载多个插件,通过 <mapped_name_of_plugin>.<name_of_parameter>
方式可以检索出特定插件的参数。举个例子,如果这个插件名字为GridBased
,我们想要指定interpolation_resolution 参数,那么Gridbased.interpolation_resolution
即可,
在 createPlan()
方法中,我们需要指定一条从start到end的路径。该方法会创建一个 nav_msgs::msg::Path
并将他返回给planner server。
1 | nav_msgs::msg::Path global_path; |
步骤二:导出插件
我们已经完成了插件的制作,现在需要将其导出,这样Planner Server才可以找到它。在ROS2中,导入和导出一个插件是通过 pluginlib
来完成的
- 代码的最后我们需要添加两行
1 | #include "pluginlib/class_list_macros.hpp" |
Pluginlib会提供一个宏 PLUGINLIB_EXPORT_CLASS
,他可以完成导出的所有工作
这两行也可以写在做在文件的最前面
- 接下来需要添加一下该描述该插件的xml文件,在根目录,创建global_planner_plugin.xml
1
2
3
4
5<library path="nav2_straightline_planner_plugin">
<class name="nav2_straightline_planner/StraightLine" type="nav2_straightline_planner::StraightLine" base_class_type="nav2_core::GlobalPlanner">
<description>This is an example plugin which produces straight path.</description>
</class>
</library>
属性信息:
library path
: 插件的名字class name
: 类名class type
: 类的类型base class
: 基类的类名description
: 插件作用的描述
- 下一步我们需要通过CMakeLists来导出插件,利用
pluginlib_export_plugin_description_file()
.函数,他可以把插件安装到share目录下
1 | pluginlib_export_plugin_description_file(nav2_core global_planner_plugin.xml) |
- 插件的描述应该被添加到
package.xml
中
1 | <export> |
- 编译,生成插件
步骤三:使用插件
我们需要去修改Nav2的配置文件,修改原来的Planner插件
1 | planner_server: |
在上面的配置文件中,我们为id为GridBased的插件 指定nav2_straightline_planner/StraightLine
插件,为了使用插件特定的参数我们可以使用 <plugin_id>.<plugin_specific_parameter>
.
步骤四:跑起来!
1 | $ ros2 launch nav2_bringup tb3_simulation_launch.py params_file:=/path/to/your_params_file.yaml |