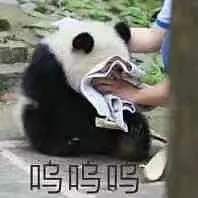
背景
Launch文件用于启动节点,服务以及执行进程。这一些行为会涉及到参数,不同的参数会有不同的行为。在描述可重用的启动文件时,可以在参数中使用替换以提供更大的灵活性。Substitutions是仅在执行启动描述期间计算的变量,可用于获取特定信息,如启动配置、环境变量,或用于计算任意Python表达式。
前提准备
你需要创建一个类型为ament_cmake
的launch_tutorial
包
使用substitutions
1. Parent launch 文件
首先我们会创建一个launch文件,他会通过适当的参数来调用其他文件,下面我们在launch文件夹下创建example_main.launch.py
1 | from launch_ros.substitutions import FindPackageShare |
在example_main.launch.py文件中,FindPackageShare
substitution 用于寻找launch_tutorial包的share文件夹,PathJoinSubstitution
则用于组合目录
1 | PathJoinSubstitution([ |
launch_arguments
字典被传入到IncludeLaunchDescription
中, TextSubstitution
substitution 用于定义new_background_r 的值
1 | launch_arguments={ |
2 Substitutions example launch file
在同一个文件下创建文件 example_substitutions.launch.py
1 | from launch_ros.actions import Node |
在文件example_substitutions.launch.py中,定义了turtlesim_ns
, use_provided_red
, 和 new_background_r
的launch configurations 。它们用于在上述变量中存储launch参数的值,并将它们传递给所需的action。这些LaunchConfiguration substitutions允许我们在launch的任何部分来获得launch参数的值。
DeclareLaunchArgument用于定义可以从上面的启动文件或控制台传递的启动参数。
1 | turtlesim_ns = LaunchConfiguration('turtlesim_ns') |
节点的turtlesim_node的namespace
被指定为turtlesim_ns
,此substitutions之前已定义过
1 | turtlesim_node = Node( |
Launching example
1 | ros2 launch launch_tutorial example_main.launch.py |
修改launch参数
在修改之前,我们需要知道可以修改什么参数
1 | ros2 launch launch_tutorial example_substitutions.launch.py --show-args |
这可以返回有什么参数,并且有什么默认值
1 | Arguments (pass arguments as '<name>:=<value>'): |
现在我们可以修改参数
1 | ros2 launch launch_tutorial example_substitutions.launch.py turtlesim_ns:='turtlesim3' use_provided_red:='True' new_background_r:=200 |